Mastering Patterns in Algorithm Design
Introduction
Have you ever tried following a recipe to bake your favorite cookies? Imagine you're a teacher guiding your students through the steps, ensuring each ingredient is added at just the right moment. This simple process is a perfect example of algorithm design in action. But what exactly is an algorithm, and how do patterns play a role in creating efficient and effective solutions?
Algorithms are everywhere—from the apps we use daily to the way our teachers organize classroom activities. They are essentially step-by-step instructions that solve problems or perform tasks. Understanding the patterns within these algorithms can make designing and implementing them much easier, whether you're coding a game, managing data, or planning a school project.
Picture this: You're organizing a classroom library. You want your students to easily find the books they're interested in without the chaos of misplaced titles. By recognizing patterns in how books are sorted—perhaps by genre, author, or color—you can create an organized and efficient system. This is a practical application of pattern recognition in algorithm design.
But why is mastering patterns so crucial for both teachers and students in the realm of informatics? Simply put, patterns help us predict and understand complex systems, making problem-solving more intuitive. When you recognize a pattern, you can apply a proven solution rather than reinventing the wheel each time you encounter a similar problem. This not only saves time but also enhances creativity and innovation.
In this article, we'll embark on a journey to explore the fascinating world of patterns in algorithm design. We'll delve into key concepts, share relatable examples, and provide practical applications that you can integrate into your teaching or learning process. Whether you're a teacher aiming to make your lessons more engaging or a student eager to enhance your computational thinking skills, understanding these patterns will empower you to tackle challenges with confidence.
So, let’s dive in and uncover how mastering patterns can transform the way we approach algorithm design, making it a seamless part of our everyday lives.
Understanding Pattern Recognition in Algorithms
At the heart of algorithm design lies pattern recognition—the ability to identify repeated sequences or regularities within data or processes. Just like recognizing that the traffic light sequence follows a predictable pattern, algorithms often rely on identifying and utilizing such patterns to function efficiently.
What is Pattern Recognition?
Pattern recognition involves detecting arrangements or sequences that recur within a given context. In algorithm design, this means observing how data behaves and finding consistent methods to address specific problems. By recognizing these patterns, we can create algorithms that are not only effective but also optimized for performance.
Example: Sorting Books in the Classroom
✍️ Example: Imagine you're sorting books in a classroom. Initially, the books are scattered randomly across the shelves. To organize them efficiently, you might notice patterns such as grouping books by genre, author, or color. By identifying and applying one of these patterns consistently, you create a system that makes it easier for students to find their desired books quickly.
This simple act of sorting utilizes pattern recognition to transform a chaotic arrangement into an orderly system, much like how algorithms operate on data to produce organized and meaningful outcomes.
Why is Pattern Recognition Important?
Recognizing patterns allows us to simplify complex problems by breaking them down into manageable parts. It enables us to apply existing solutions to new but similar challenges, fostering a more intuitive approach to problem-solving. In the context of computational thinking, pattern recognition is a foundational skill that underpins other elements like abstraction and decomposition.
🔍 Fun Fact: The concept of pattern recognition in algorithms is inspired by how the human brain processes information, allowing us to quickly identify and respond to familiar stimuli.
How to Develop Pattern Recognition Skills
Enhancing your pattern recognition skills involves practice and exposure to various problem-solving scenarios. Here are a few strategies:
- Practice Regularly: Engage with different types of problems to spot recurring themes and solutions.
- Analyze Existing Algorithms: Study how established algorithms use patterns to achieve their objectives.
- Collaborate and Discuss: Working with others can help you see different perspectives and identify patterns you might have missed.
📘 Tip: Incorporate pattern recognition activities into your lessons, such as identifying sequences in number patterns or analyzing the structure of different algorithms.
Key Takeaways
- Pattern recognition is the ability to identify recurring sequences or arrangements within data or processes.
- Recognizing patterns simplifies complex problems, making algorithm design more intuitive and efficient.
- Developing pattern recognition skills involves regular practice, analyzing existing solutions, and collaborative learning.
Decomposition and Abstraction
Moving beyond pattern recognition, two essential concepts in algorithm design are decomposition and abstraction. These techniques help us break down complex problems into smaller, more manageable parts and simplify the overarching structure of our solutions.
Decomposition: Breaking Down the Problem
Decomposition involves dividing a large, complex problem into smaller, more manageable sub-problems. This approach makes it easier to tackle each part individually, ensuring that the overall solution is well-organized and effective.
Example: Planning a School Event
Empower Digital Minds Through Bebras
1,400 Schools
Enable every school in Armenia to participate in Bebras, transforming informatics education from a subject into an exciting journey of discovery.
380,000 Students
Give every student the chance to develop crucial computational thinking skills through Bebras challenges, preparing them for success in our digital world.
Help us bring the exciting world of computational thinking to every Armenian school through the Bebras Competition. Your support doesn't just fund a contest - it ignites curiosity in informatics and builds problem-solving skills that last a lifetime.
I Want to Donate Now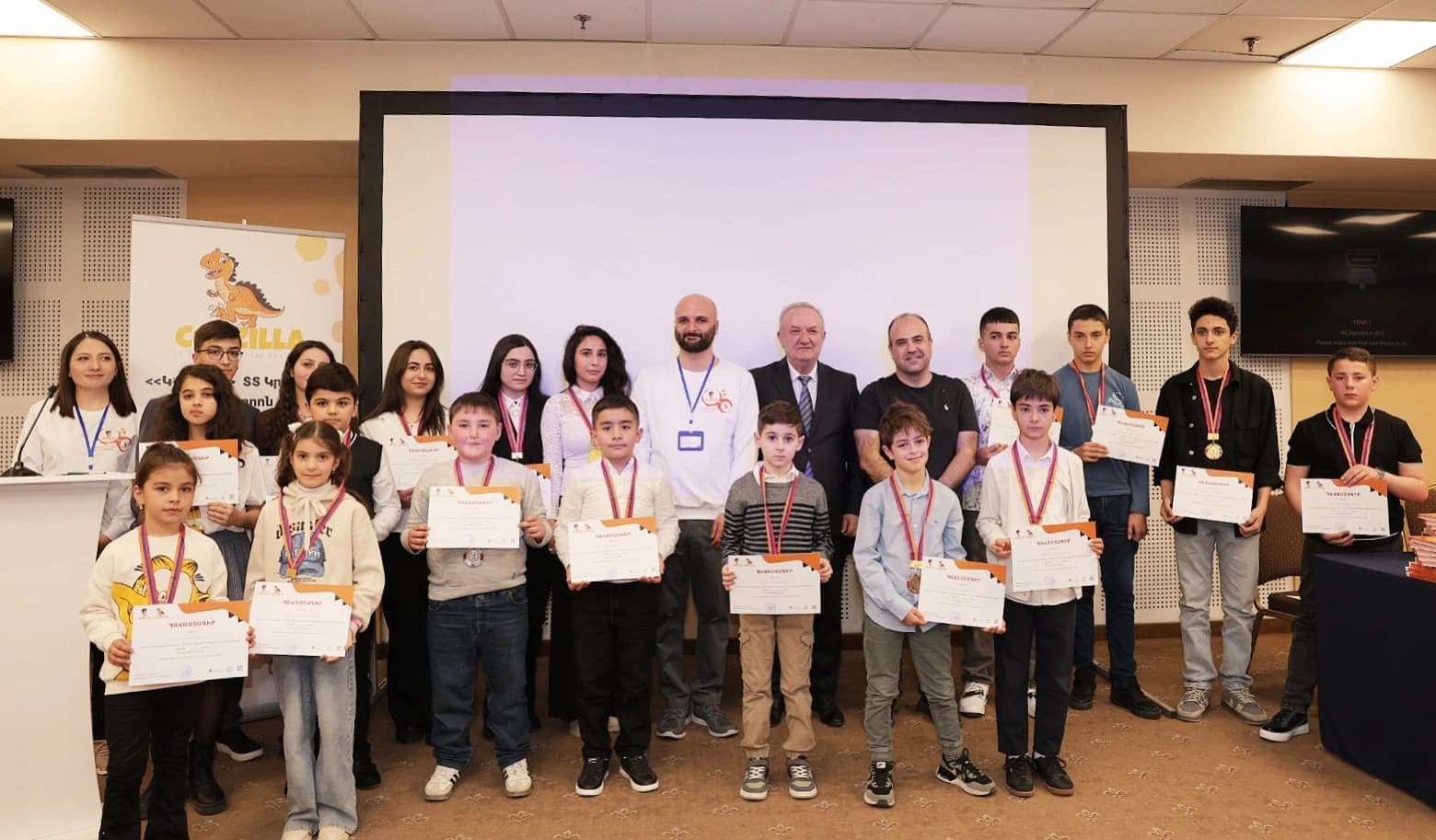
✍️ Example: Suppose you're planning a school event like a science fair. At first glance, it might seem overwhelming with tasks like arranging venues, coordinating with participants, setting up displays, and managing logistics. By decomposing the event planning into smaller tasks—such as venue selection, participant registration, display setup, and logistics—you can address each component systematically, ensuring nothing is overlooked.
This methodical breakdown helps in assigning responsibilities, tracking progress, and ensuring that each aspect of the event is handled efficiently.
Abstraction: Simplifying Complex Systems
Abstraction involves focusing on the essential features of a problem while ignoring the irrelevant details. By doing so, we can create a simplified representation of a complex system, making it easier to understand and work with.
Example: Designing a School Timetable
✍️ Example: Creating a school timetable involves numerous details like class schedules, teacher availability, room assignments, and extracurricular activities. To abstract this complexity, you might focus solely on time slots and subject allocations, temporarily setting aside specifics like room numbers or teacher preferences. This simplified model allows you to organize the timetable effectively before incorporating additional details.
Abstraction helps in managing complexity by allowing you to concentrate on what's crucial for solving the problem at hand, ensuring that the algorithm remains focused and efficient.
💡 Insight: Combining decomposition and abstraction enables you to handle complex problems logically and systematically, making algorithm design more approachable and less daunting.
Applying Decomposition and Abstraction Together
When designing algorithms, using both decomposition and abstraction can significantly enhance your problem-solving process. By breaking down the problem and simplifying it, you create a clear roadmap for developing effective solutions.
📘 Tip: Encourage students to use decomposition and abstraction in their projects. For instance, when coding a simple game, they can decompose the game mechanics into smaller functionalities (like player movement, scoring, and levels) and abstract these components to focus on the main gameplay without getting bogged down by intricate details initially.
Key Takeaways
- Decomposition involves breaking down complex problems into smaller, manageable sub-problems.
- Abstraction focuses on the essential features, simplifying complex systems by ignoring irrelevant details.
- Using decomposition and abstraction together streamlines the algorithm design process, making it more efficient and effective.
Algorithmic Paradigms: Building Block Approaches
Once we've recognized patterns and employed decomposition and abstraction, the next step in mastering algorithm design is understanding different algorithmic paradigms. These paradigms serve as foundational strategies or templates that guide how we approach and solve problems.
What are Algorithmic Paradigms?
Algorithmic paradigms are general approaches or methodologies used to solve a wide range of problems. They provide a structured way to think about problem-solving, ensuring that solutions are both effective and efficient.
Common Paradigms
- Iterative Approach: Repeating a set of instructions until a condition is met.
- Recursive Approach: Solving a problem by breaking it down into smaller instances of the same problem.
- Divide and Conquer: Dividing the problem into independent sub-problems, solving each, and combining the results.
- Greedy Algorithms: Making the locally optimal choice at each step with the hope of finding the global optimum.
- Dynamic Programming: Solving complex problems by breaking them down into simpler sub-problems and storing the results of these sub-problems to avoid redundant computations.
Example: Implementing a Sorting Algorithm
✍️ Example: Let's consider sorting a list of student names alphabetically. You could use different algorithmic paradigms to achieve this:
- Iterative Approach: Repeatedly compare and swap adjacent names until the entire list is sorted.
- Divide and Conquer: Split the list into smaller sub-lists, sort each independently, and then merge them back together in order.
- Greedy Algorithm: At each step, pick the smallest remaining name and place it in the next position of the sorted list.
Each paradigm offers a unique way to approach the sorting problem, with varying levels of efficiency and complexity.
💡 Insight: Understanding different algorithmic paradigms equips you with a toolbox of strategies, allowing you to select the most appropriate one based on the problem's nature and constraints.
Choosing the Right Paradigm
Selecting the appropriate algorithmic paradigm depends on several factors:
- Problem Characteristics: Some problems are naturally suited to certain paradigms. For example, recursive approaches are ideal for problems that can be broken down into similar sub-problems.
- Efficiency Requirements: Depending on the desired performance, certain paradigms may offer faster or more resource-efficient solutions.
- Complexity: Simpler paradigms might be preferable for straightforward problems, while more complex ones are reserved for intricate challenges.
📘 Tip: When introducing algorithmic paradigms to students, use real-life situations they can relate to. For instance, planning a daily schedule can illustrate the greedy algorithm by prioritizing the most important tasks first.
Key Takeaways
Empower Digital Minds Through Bebras
1,400 Schools
Enable every school in Armenia to participate in Bebras, transforming informatics education from a subject into an exciting journey of discovery.
380,000 Students
Give every student the chance to develop crucial computational thinking skills through Bebras challenges, preparing them for success in our digital world.
Help us bring the exciting world of computational thinking to every Armenian school through the Bebras Competition. Your support doesn't just fund a contest - it ignites curiosity in informatics and builds problem-solving skills that last a lifetime.
I Want to Donate Now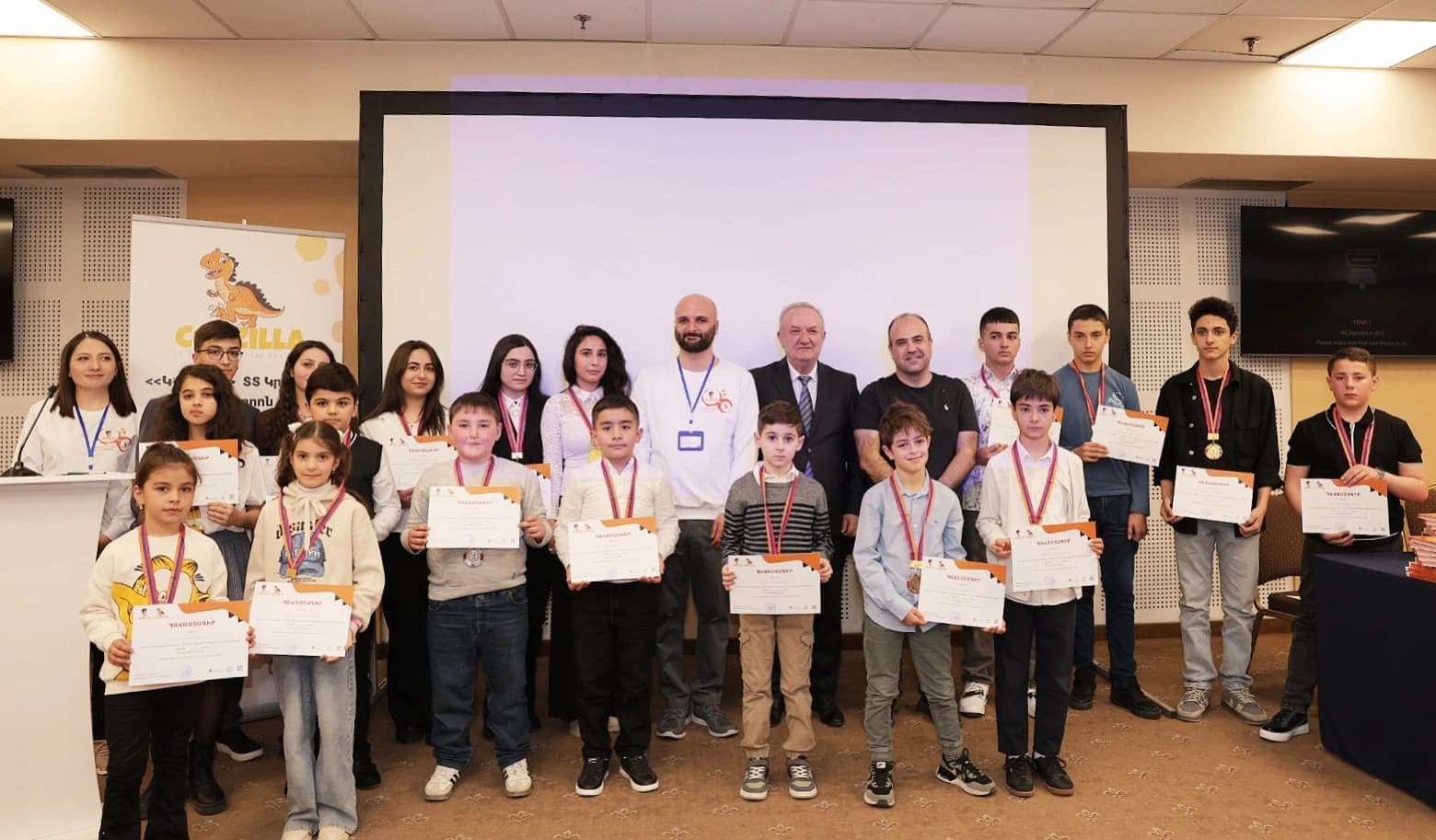
- Algorithmic paradigms are foundational strategies used to approach and solve problems systematically.
- Common paradigms include iterative, recursive, divide and conquer, greedy algorithms, and dynamic programming.
- Choosing the right paradigm depends on the problem's characteristics, efficiency requirements, and complexity.
Optimization and Efficiency in Algorithms
Designing algorithms isn't just about solving problems—it's also about doing so in the most efficient way possible. Optimization and efficiency are crucial aspects of algorithm design that ensure your solutions are not only correct but also performant and scalable.
Understanding Optimization
Optimization involves refining an algorithm to make it work more efficiently. This can mean reducing the time it takes to execute, decreasing the amount of memory it uses, or minimizing other resources it consumes.
Example: Streamlining Classroom Assignments
✍️ Example: Suppose you're assigning students to different classroom activities. Initially, you might use a simple method where you manually allocate students based on their preferences. However, this can become time-consuming as the number of students grows. By optimizing the assignment process—perhaps by using a matching algorithm that automatically pairs students with activities based on their preferences—you can save time and reduce the chance of errors.
This optimization ensures that the assignment process is scalable and efficient, especially in larger classrooms or more complex scenarios.
Measuring Efficiency: Time and Space Complexity
Two primary metrics used to assess an algorithm's efficiency are time complexity and space complexity.
- Time Complexity: Refers to the amount of time an algorithm takes to complete as a function of the input size.
- Space Complexity: Refers to the amount of memory an algorithm uses relative to the input size.
Understanding these complexities helps in evaluating and comparing different algorithms, ensuring that the chosen solution meets the necessary performance criteria.
🔍 Fun Fact: The concept of Big O notation is commonly used to describe the upper bound of an algorithm's time or space complexity, providing a high-level understanding of its efficiency.
Strategies for Optimization
Here are some common strategies to optimize algorithms:
- Eliminate Redundancies: Remove unnecessary steps or calculations that don't contribute to the final outcome.
- Improve Data Structures: Choosing the right data structure can significantly enhance an algorithm's performance.
- Leverage Efficient Paradigms: As discussed earlier, selecting the most appropriate algorithmic paradigm can lead to more efficient solutions.
- Memoization: Storing the results of expensive function calls and reusing them when the same inputs occur again.
📘 Tip: Encourage students to analyze their algorithms for potential optimizations. This not only improves performance but also deepens their understanding of how algorithms work under the hood.
Key Takeaways
- Optimization enhances an algorithm's efficiency by reducing its resource consumption.
- Time and space complexity are critical metrics for evaluating an algorithm's performance.
- Strategies like eliminating redundancies, improving data structures, and leveraging efficient paradigms are essential for optimizing algorithms.
Practical Applications: Bringing Concepts to Life
Understanding algorithm design and the role of patterns is one thing, but applying these concepts to real-world scenarios makes the learning process engaging and meaningful. Let’s explore some practical applications that demonstrate how these principles come together in everyday situations.
Example 1: Organizing Digital Classroom Resources
✍️ Example: Imagine you're managing a digital classroom with various resources like lecture notes, assignments, and multimedia content. To keep everything organized, you can apply algorithm design principles:
- Pattern Recognition: Identify common themes or subjects within your resources.
- Decomposition: Break down resources into categories such as subjects, topics, and formats.
- Algorithmic Paradigm: Use a sorting algorithm to arrange resources alphabetically or by date.
- Optimization: Implement a search function that quickly retrieves resources based on keywords, enhancing efficiency.
By applying these patterns, you create a well-structured and easily navigable digital classroom environment, benefiting both teachers and students.
Example 2: Navigating Educational Apps
✍️ Example: Educational apps often rely on underlying algorithms to provide personalized learning experiences. For instance, a math learning app might use:
- Pattern Recognition: Identify areas where a student struggles based on their answers.
- Decomposition: Break down math topics into sub-topics like algebra, geometry, and calculus.
- Algorithmic Paradigm: Implement a dynamic scheduling algorithm that adjusts the difficulty level of questions based on performance.
- Optimization: Ensure the app runs smoothly on various devices by optimizing code for speed and memory usage.
Empower Digital Minds Through Bebras
1,400 Schools
Enable every school in Armenia to participate in Bebras, transforming informatics education from a subject into an exciting journey of discovery.
380,000 Students
Give every student the chance to develop crucial computational thinking skills through Bebras challenges, preparing them for success in our digital world.
Help us bring the exciting world of computational thinking to every Armenian school through the Bebras Competition. Your support doesn't just fund a contest - it ignites curiosity in informatics and builds problem-solving skills that last a lifetime.
I Want to Donate Now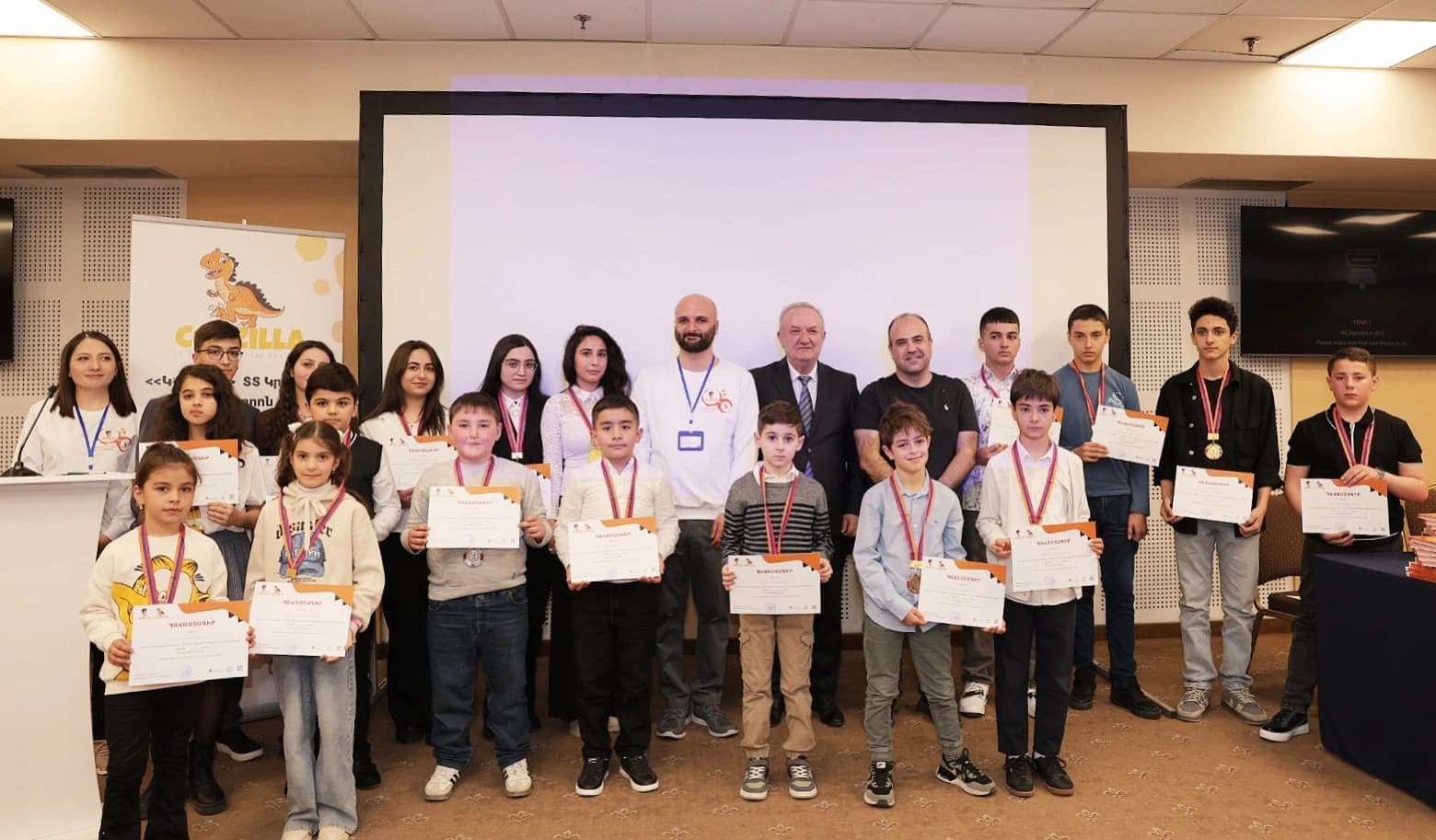
These applications demonstrate how algorithm design patterns enhance user experience and learning outcomes in educational technology.
💡 Insight: Incorporating real-world examples like these into your teaching can make abstract concepts more tangible and relatable for students.
Example 3: Managing Group Projects
✍️ Example: Coordinating group projects in a classroom can benefit from algorithmic thinking:
- Pattern Recognition: Observe common challenges in past group projects, such as unequal task distribution.
- Decomposition: Divide the project into specific roles and tasks like research, presentation, and documentation.
- Algorithmic Paradigm: Use a scheduling algorithm to allocate tasks based on each student’s strengths and availability.
- Optimization: Implement a feedback loop where students can adjust their tasks if needed, ensuring the project stays on track.
This structured approach fosters collaboration, ensures fairness, and enhances the overall efficiency of group work.
✨ Mnemonic: Remember POGO—Pattern recognition, Optimization, Growth through decomposition, and Ongoing evaluation—to apply algorithm design principles effectively.
Key Takeaways
- Practical applications of algorithm design make learning more engaging and relevant.
- Organizing digital resources, enhancing educational apps, and managing group projects are just a few examples where these concepts can be applied.
- Using real-world scenarios helps students grasp abstract concepts and see their value in everyday life.
Conclusion
As we've journeyed through the intricate landscape of algorithm design and the pivotal role of patterns, it's clear that these concepts are not just abstract theories but practical tools that enhance our daily lives. From organizing classroom resources to developing intelligent educational apps, the principles of pattern recognition, decomposition, abstraction, and optimization empower both educators and students to solve problems efficiently and creatively.
Imagine you’re standing at the crossroads of technology and education, armed with the knowledge of algorithmic thinking. You have the power to design solutions that streamline processes, personalize learning experiences, and foster a collaborative environment. By mastering these patterns, you're not only enhancing your computational skills but also equipping yourself and your students with the ability to navigate and shape the ever-evolving digital landscape.
Moreover, these skills transcend the boundaries of informatics. They cultivate critical thinking, logical reasoning, and a structured approach to problem-solving—qualities that are invaluable in any field. As educators, incorporating these concepts into your teaching fosters a classroom environment that encourages curiosity, innovation, and resilience. For students, understanding these patterns lays a strong foundation for future endeavors in technology, science, and beyond.
So, let’s embrace the patterns in algorithm design and harness their potential to create more organized, efficient, and impactful solutions. Whether you're coding a new project, managing classroom activities, or simply looking to enhance your problem-solving toolkit, these principles are your allies in making the complex simple and the impossible achievable.
Challenge for You: Think of a recurring problem in your teaching or learning environment. How can you apply the patterns of algorithm design to create a more efficient and effective solution? Share your ideas and let’s build a community of innovative problem-solvers together!
Key Takeaways
- Algorithm design patterns are practical tools that enhance problem-solving in everyday scenarios.
- Mastering these patterns fosters critical thinking, logical reasoning, and a structured approach to challenges.
- Applying algorithmic principles in education empowers both teachers and students to create efficient and impactful solutions.
Want to Learn More?
- 📚 Introduction to Algorithms by Cormen, Leiserson, Rivest, and Stein
- 🖥️ Khan Academy's Algorithms Course
- 🎓 Coursera's Algorithm Specialization
- 🌐 GeeksforGeeks Algorithm Tutorials
Final Takeaway
Embracing the patterns in algorithm design transforms how we approach and solve problems, making complex challenges manageable and solutions more effective. By fostering a deeper understanding of these concepts, we not only enhance our technical skills but also cultivate a mindset geared towards innovation and continuous improvement. So, let’s continue exploring, experimenting, and applying these patterns, turning every challenge into an opportunity for growth and discovery.