Debugging Techniques for Algorithm Success
Introduction
Have you ever spent hours trying to solve a tricky puzzle, only to realize a small mistake was holding you back? Imagine you're working on a complex math problem or assembling a model—it’s easy to overlook that one tiny detail that changes everything. In the world of computer science, this scenario plays out every day through the process of debugging.
Debugging is like being a detective in the digital realm, where your mission is to find and fix errors in code to ensure algorithms run smoothly. Whether you're a teacher guiding students through their first lines of code or a student crafting your own programs, understanding effective debugging techniques is essential for success in algorithmic thinking.
But why is debugging so crucial? Consider this: even the most brilliant algorithm can fail if it contains bugs—those pesky errors that prevent your code from working as intended. Debugging not only helps in identifying these issues but also strengthens your problem-solving skills, making you a more proficient coder.
Let's dive into the fascinating world of debugging, exploring strategies that make error-finding less daunting and more manageable. From understanding common types of bugs to employing systematic approaches for troubleshooting, this article will equip you with the tools needed to navigate the challenges of algorithm development.
Picture this: you're in the middle of a coding project, and suddenly your program doesn't behave as expected. It’s frustrating, right? But with the right debugging techniques, what seems like a roadblock becomes an opportunity to learn and improve your coding prowess. Together, we’ll explore how to transform these challenges into stepping stones toward algorithmic success.
So, let’s embark on this journey to demystify debugging. Whether you’re a teacher looking for ways to support your students or a student aiming to enhance your coding skills, mastering debugging techniques will empower you to create more reliable and efficient algorithms. Ready to become a debugging expert? Let’s get started!
Understanding Bugs in Algorithms
Before diving into debugging techniques, it’s essential to understand what bugs are and how they manifest in algorithms. Bugs are errors or flaws in a program that prevent it from functioning correctly. They can occur for various reasons, such as syntax errors, logical mistakes, or runtime issues.
Types of Bugs
There are several types of bugs that you might encounter:
-
Syntax Errors: These are mistakes in the code's structure, such as missing semicolons or unmatched parentheses. They prevent the code from compiling or running.
-
Logical Errors: These occur when the code doesn’t perform as intended, even though it runs without crashing. For example, using the wrong formula in a calculation.
-
Runtime Errors: These happen during the execution of the program, such as dividing by zero or accessing invalid memory locations.
Common Causes of Bugs
Understanding the root causes of bugs can help in preventing and fixing them:
-
Human Error: Simple typos or miscalculations can introduce bugs.
-
Miscommunication: Misunderstanding the problem requirements can lead to incorrect implementations.
-
Complexity: As algorithms become more complex, the likelihood of introducing bugs increases.
-
Incomplete Testing: Failing to test all possible scenarios can leave bugs undiscovered.
The Impact of Bugs on Algorithm Success
Bugs can severely impact the effectiveness of an algorithm. They can lead to incorrect results, inefficient performance, or even complete failure of the program. For students and teachers, encountering bugs is a valuable learning opportunity. It encourages critical thinking and reinforces the importance of precision in programming.
💡 Insight: Embracing bugs as learning tools can transform frustration into growth, enhancing both coding skills and problem-solving abilities.
✍️ Example: Imagine you're creating a sorting algorithm for a classroom project. A single misplaced comparison operator causes the entire sorting process to fail, leaving the list unordered. By identifying and correcting this logical error, you not only fix the algorithm but also gain a deeper understanding of how sorting mechanisms work.
Key Takeaways
- Bugs are errors in code that can be syntax, logical, or runtime-related.
- Common causes include human error, miscommunication, complexity, and incomplete testing.
- Understanding the types and causes of bugs is the first step toward effective debugging.
Try This!
Quiz Question: What type of bug occurs when the code doesn't perform as intended but runs without crashing?
- A) Syntax Error
- B) Logical Error
- C) Runtime Error
- D) Compilation Error
Answer: B) Logical Error
Empower Digital Minds Through Bebras
1,400 Schools
Enable every school in Armenia to participate in Bebras, transforming informatics education from a subject into an exciting journey of discovery.
380,000 Students
Give every student the chance to develop crucial computational thinking skills through Bebras challenges, preparing them for success in our digital world.
Help us bring the exciting world of computational thinking to every Armenian school through the Bebras Competition. Your support doesn't just fund a contest - it ignites curiosity in informatics and builds problem-solving skills that last a lifetime.
I Want to Donate Now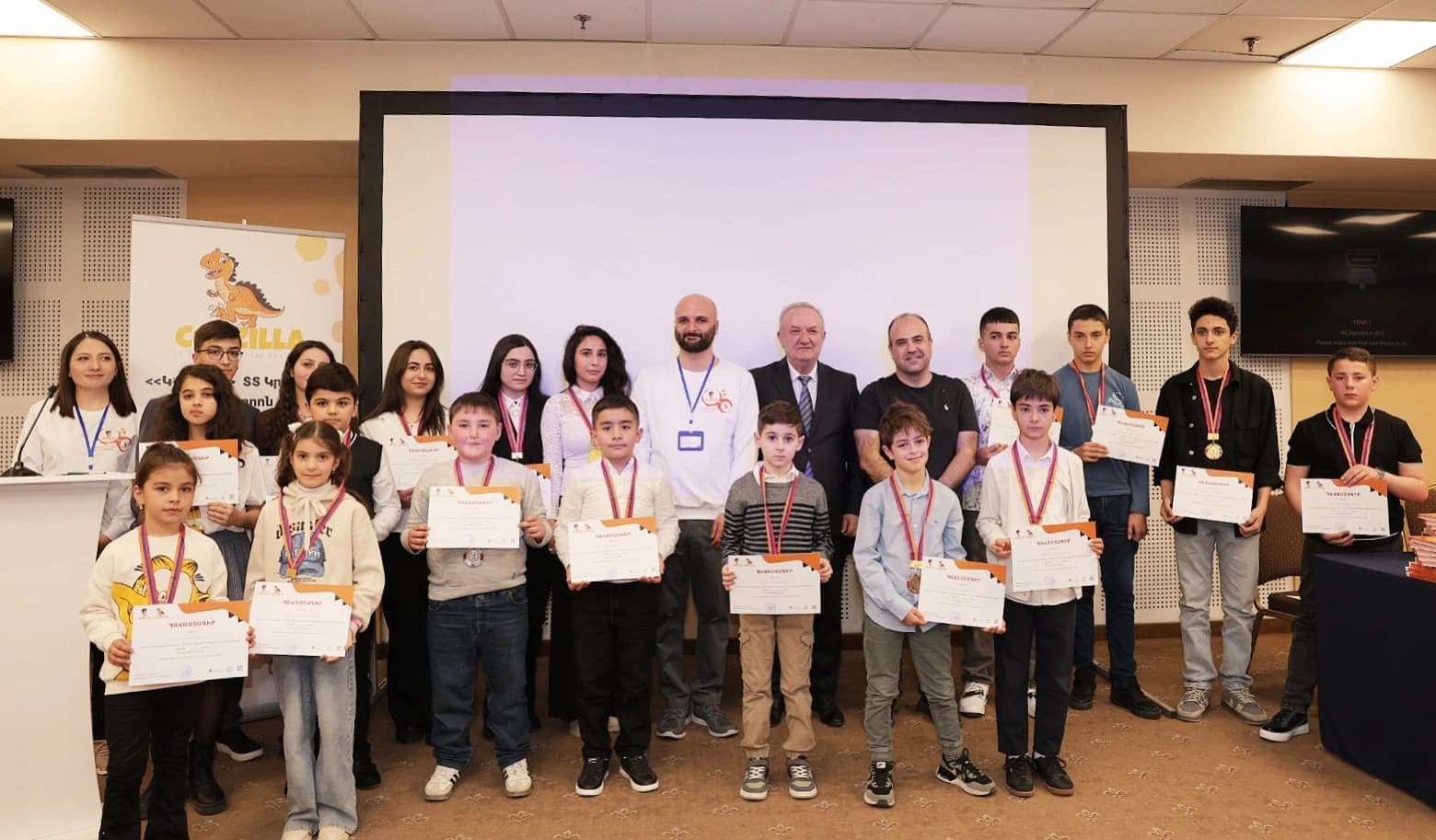
Systematic Debugging Approaches
Now that we have a grasp on what bugs are, let’s explore systematic approaches to debugging. A structured method can make the debugging process more efficient and less overwhelming.
The Debugging Process
A typical debugging process involves the following steps:
- Identify the Problem: Determine what is not working as expected.
- Reproduce the Error: Consistently replicate the issue to understand its behavior.
- Isolate the Source: Narrow down the code section where the bug resides.
- Analyze the Cause: Understand why the bug is occurring.
- Fix the Bug: Implement a solution to correct the error.
- Test the Solution: Ensure that the fix resolves the issue without introducing new bugs.
Techniques for Effective Debugging
Several techniques can aid in the debugging process:
-
Rubber Duck Debugging: Explaining your code line-by-line to an inanimate object (like a rubber duck) to identify errors through articulation.
-
Code Reviews: Having others examine your code can provide new perspectives and catch mistakes you might have missed.
-
Using Debugging Tools: Integrated Development Environments (IDEs) often come with built-in debugging tools that allow you to step through code, inspect variables, and monitor program execution.
-
Print Statements: Inserting print statements can help track the flow of execution and the state of variables at various points in the code.
The Importance of a Systematic Approach
A systematic approach prevents random guesswork and makes the debugging process more efficient. It helps in breaking down complex problems into manageable parts, ensuring that you address each potential issue methodically rather than overlooking critical aspects.
💡 Insight: Adopting a structured debugging process can significantly reduce the time and frustration associated with finding and fixing bugs.
✍️ Example: Suppose you're working on an algorithm that calculates the average of a list of numbers. However, the result is consistently lower than expected. By following a systematic approach, you first identify the problem, reproduce it by using test cases, isolate the loop that sums the numbers, analyze the averaging step, discover that you forgot to divide by the correct count, fix the calculation, and finally test to ensure the average is now accurate.
Key Takeaways
- A systematic debugging process involves identifying, reproducing, isolating, analyzing, fixing, and testing.
- Techniques like rubber duck debugging, code reviews, debugging tools, and print statements aid in effective debugging.
- A structured approach enhances efficiency and effectiveness in resolving bugs.
Try This!
Self-Reflection Prompt: Think about a time when you encountered a bug. How did you approach solving it? Was your method systematic, or did you try random fixes?
Common Debugging Strategies
Beyond the general process, specific strategies can help in tackling different types of bugs. Let’s delve into some of the most effective debugging strategies used by programmers.
Divide and Conquer
This strategy involves breaking down the code into smaller sections and testing each part independently. By isolating sections, you can narrow down the location of the bug more quickly.
- Implementation: Use functions or modules to compartmentalize code. Test each function separately to ensure it behaves correctly before integrating it into the larger program.
Backtracking
Backtracking involves retracing your steps to identify where things went wrong. This method is particularly useful when you know that the code was working previously.
- Implementation: Identify the point at which the code starts failing and review the changes made since the last known working state.
Binary Search Method
Empower Digital Minds Through Bebras
1,400 Schools
Enable every school in Armenia to participate in Bebras, transforming informatics education from a subject into an exciting journey of discovery.
380,000 Students
Give every student the chance to develop crucial computational thinking skills through Bebras challenges, preparing them for success in our digital world.
Help us bring the exciting world of computational thinking to every Armenian school through the Bebras Competition. Your support doesn't just fund a contest - it ignites curiosity in informatics and builds problem-solving skills that last a lifetime.
I Want to Donate Now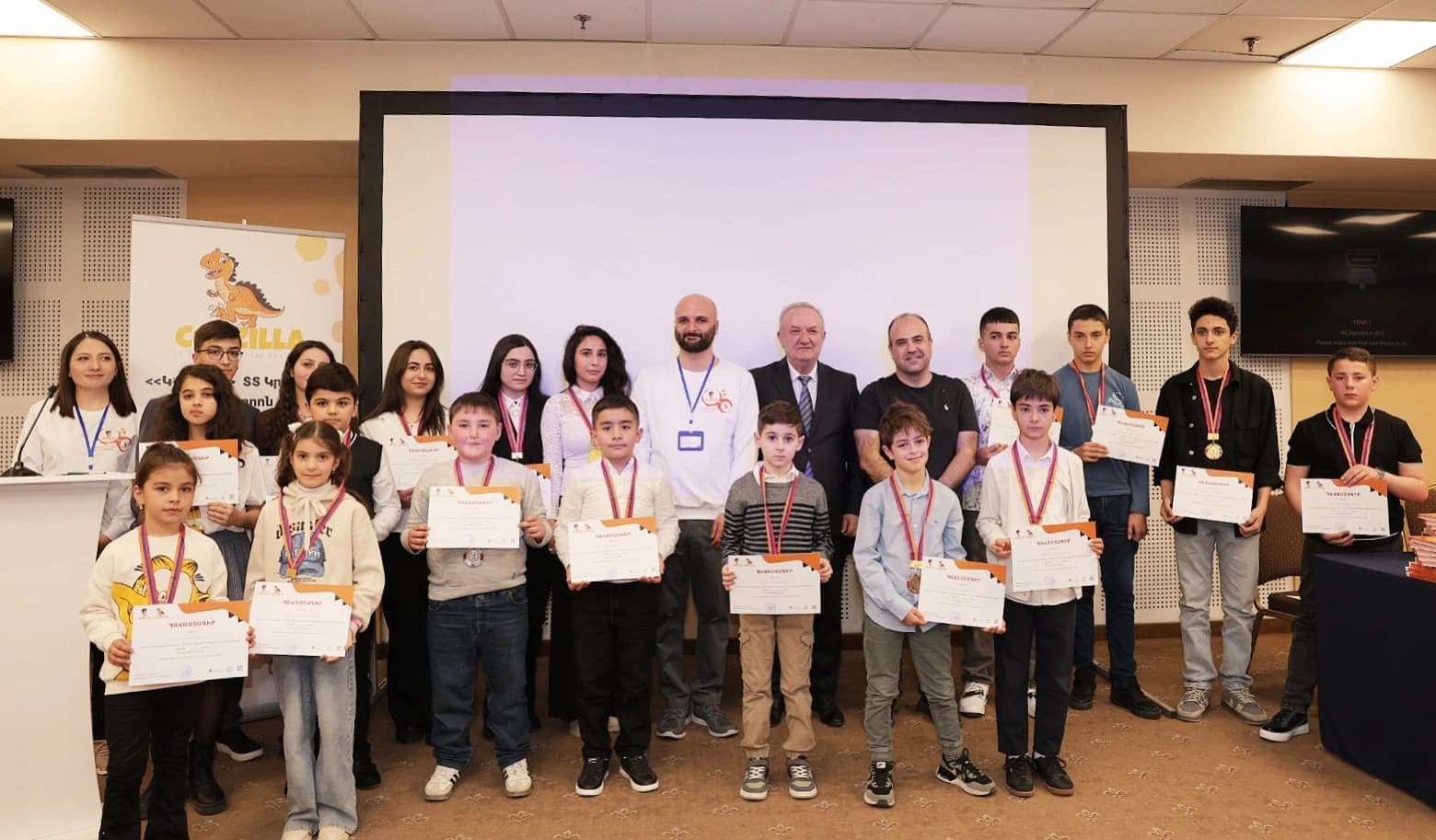
Similar to the divide and conquer approach, the binary search method involves repeatedly dividing the codebase in half to locate the bug. This is efficient for large codebases where pinpointing the issue can be challenging.
- Implementation: Comment out or disable sections of code to see if the bug persists. If it doesn’t, the issue lies in the disabled section. Repeat the process until the exact location is found.
Importance of Choosing the Right Strategy
Different bugs may require different strategies. Understanding which approach to apply can save time and reduce frustration. It’s often beneficial to combine multiple strategies for more complex issues.
💡 Insight: Flexibility in choosing and combining debugging strategies can enhance problem-solving efficiency and lead to quicker resolutions.
✍️ Example: Imagine you’re debugging a program that processes student grades. The final output is incorrect, but earlier steps seem fine. By applying the divide and conquer strategy, you isolate the grade calculation function and discover that it incorrectly handles edge cases, such as rounding errors. Fixing this function resolves the issue without overhauling the entire program.
Key Takeaways
- Divide and Conquer: Break down the code and test each section independently.
- Backtracking: Retrace steps to identify when the bug was introduced.
- Binary Search Method: Systematically narrow down the codebase to locate the bug.
- Choosing the right strategy depends on the nature and complexity of the bug.
Try This!
Quiz Question: Which debugging strategy involves splitting the codebase and testing each half to locate the bug?
- A) Backtracking
- B) Divide and Conquer
- C) Binary Search Method
- D) Rubber Duck Debugging
Answer: C) Binary Search Method
Tools and Techniques for Debugging
While strategies are essential, having the right tools at your disposal can make the debugging process more efficient and less time-consuming. Let’s explore some of the most effective tools and techniques used by programmers.
Integrated Development Environment (IDE) Debuggers
Most modern IDEs come equipped with powerful debugging tools that allow you to:
- Set Breakpoints: Pause the execution of your program at specific lines of code.
- Step Through Code: Execute your program one line at a time to observe the flow and state of variables.
- Inspect Variables: View and modify the values of variables in real-time.
- Evaluate Expressions: Test expressions and evaluate code snippets during execution.
Version Control Systems
Version control systems like Git allow you to:
- Track Changes: Keep a history of changes made to the codebase.
- Revert to Previous States: Undo changes that introduced bugs.
- Collaborate Efficiently: Work with others without overwriting each other's work.
Static Code Analyzers
These tools analyze your code for potential errors without executing it. They can detect:
- Syntax Errors: Highlight mistakes in the code structure.
- Potential Bugs: Identify code that could lead to runtime errors.
- Code Smells: Suggest improvements for better code quality and maintainability.
Logging
Implementing logging in your code allows you to:
- Record Execution Flow: Keep track of the sequence in which parts of your code are executed.
- Monitor Variable States: Log the values of variables at different points.
- Diagnose Issues: Use logs to pinpoint where things go wrong.
✨ Mnemonic: DVL – Debuggers, Version control, Logging – the three pillars of effective debugging.
Key Takeaways
- IDEs offer built-in tools for setting breakpoints, stepping through code, and inspecting variables.
- Version Control Systems like Git help track changes, revert to previous states, and facilitate collaboration.
- Static Code Analyzers detect syntax errors, potential bugs, and code smells.
- Logging provides insights into the execution flow and variable states, aiding in diagnosing issues.
Try This!
Empower Digital Minds Through Bebras
1,400 Schools
Enable every school in Armenia to participate in Bebras, transforming informatics education from a subject into an exciting journey of discovery.
380,000 Students
Give every student the chance to develop crucial computational thinking skills through Bebras challenges, preparing them for success in our digital world.
Help us bring the exciting world of computational thinking to every Armenian school through the Bebras Competition. Your support doesn't just fund a contest - it ignites curiosity in informatics and builds problem-solving skills that last a lifetime.
I Want to Donate Now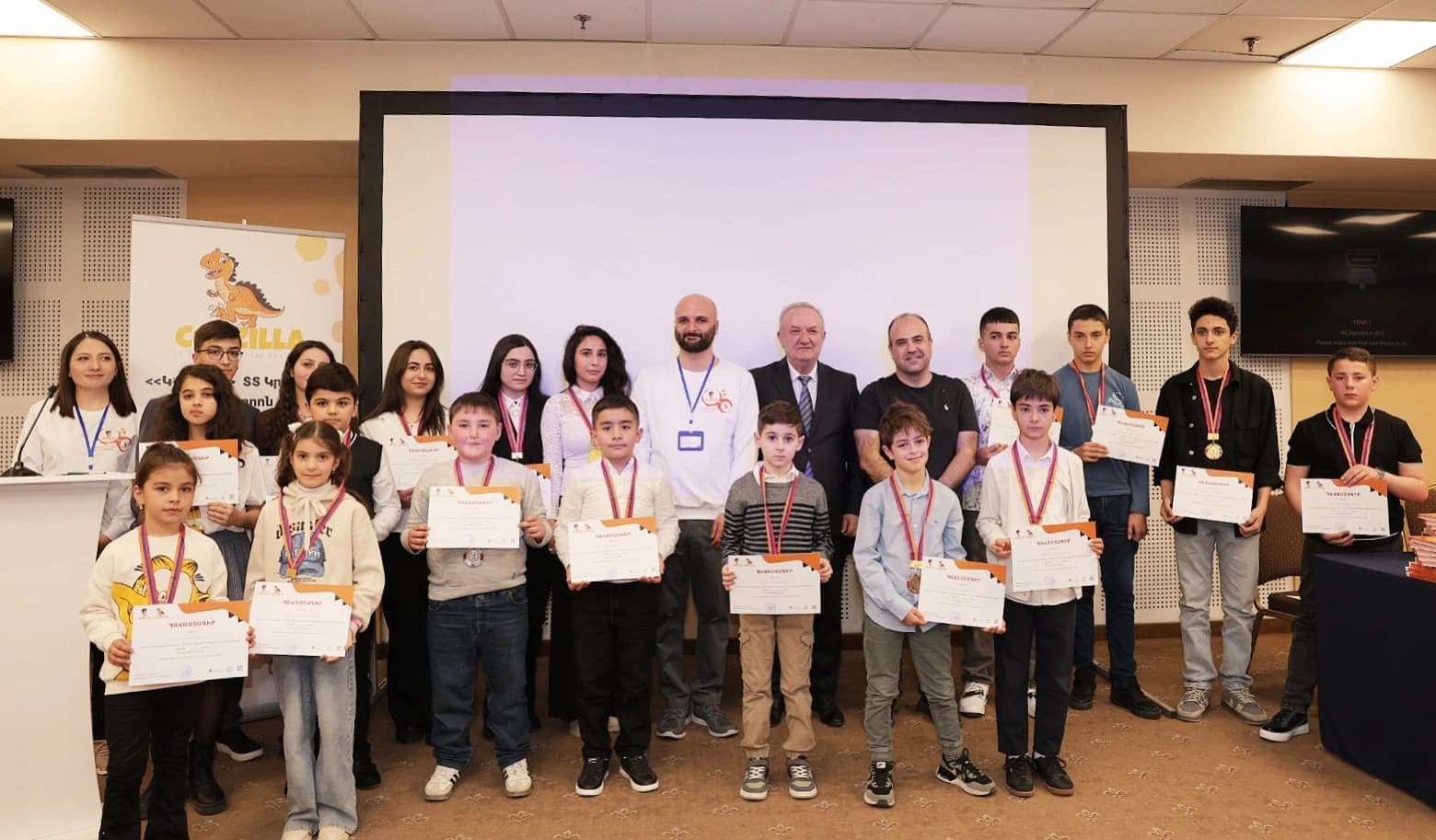
Self-Reflection Prompt: Which debugging tool do you find most useful, and how has it helped you in your coding projects?
Best Practices for Preventing Bugs
While debugging is essential, it's even better to prevent bugs from occurring in the first place. Adopting best practices can significantly reduce the number of errors in your code.
Write Clear and Readable Code
Clear code is easier to understand and maintain, reducing the likelihood of introducing bugs.
- Use Meaningful Variable Names: Choose descriptive names that convey the purpose of the variable.
- Consistent Indentation: Maintain consistent formatting for better readability.
- Comment Your Code: Explain complex sections to aid in understanding and future maintenance.
Plan Before Coding
Taking the time to plan your algorithm can help identify potential issues before writing any code.
- Pseudocode: Outline the logic in plain language before translating it into code.
- Flowcharts: Visualize the flow of your algorithm to spot logical errors early.
Test Regularly
Frequent testing allows you to catch bugs early in the development process.
- Unit Testing: Test individual components of your code to ensure they work correctly.
- Integration Testing: Verify that different parts of the system work together seamlessly.
- Edge Case Testing: Test scenarios that are unusual or extreme to ensure your code handles them gracefully.
Practice Code Reviews
Having others review your code can uncover mistakes you might have missed and provide valuable feedback.
💡 Insight: Preventing bugs through best practices not only saves time but also leads to more robust and maintainable code.
✍️ Example: Consider a student project where the goal is to create a basic calendar application. By writing clear code with meaningful variable names, planning the app’s features using pseudocode, and testing each component thoroughly, the student minimizes the chances of encountering significant bugs. Additionally, having a peer review the code can help catch any overlooked errors, ensuring a smoother development process.
Key Takeaways
- Writing clear and readable code makes it easier to understand and maintain, reducing bugs.
- Planning algorithms with pseudocode or flowcharts helps identify potential issues early.
- Regular testing, including unit, integration, and edge case testing, catches bugs early.
- Code reviews with peers provide fresh perspectives and help uncover hidden errors.
Try This!
Quiz Question: Which practice involves outlining your algorithm in plain language before coding?
- A) Code Review
- B) Pseudocode
- C) Logging
- D) Unit Testing
Answer: B) Pseudocode
Conclusion
Debugging is an integral part of the coding journey, shaping not just the functionality of your algorithms but also enhancing your problem-solving skills. By understanding the types of bugs, adopting systematic approaches, utilizing the right tools, and following best practices, you transform debugging from a daunting task into a manageable and even enjoyable process.
Every bug you encounter is an opportunity to learn and grow as a programmer. Embracing these challenges fosters a deeper comprehension of algorithmic thinking, enabling you to create more efficient and reliable code. Whether you're a teacher guiding students through their first programming steps or a student honing your coding abilities, mastering debugging techniques is pivotal to achieving algorithmic success.
As you continue to develop your coding projects, remember that debugging is not just about fixing errors—it's about building resilience and refining your analytical skills. So next time you face a bug, approach it with curiosity and determination, knowing that each fix brings you one step closer to mastering the art of programming.
Challenge: Think of a recent coding project where you encountered a bug. Reflect on the debugging techniques you used. How could applying the strategies discussed in this article improve your approach next time?
Want to Learn More?
Empower Digital Minds Through Bebras
1,400 Schools
Enable every school in Armenia to participate in Bebras, transforming informatics education from a subject into an exciting journey of discovery.
380,000 Students
Give every student the chance to develop crucial computational thinking skills through Bebras challenges, preparing them for success in our digital world.
Help us bring the exciting world of computational thinking to every Armenian school through the Bebras Competition. Your support doesn't just fund a contest - it ignites curiosity in informatics and builds problem-solving skills that last a lifetime.
I Want to Donate Now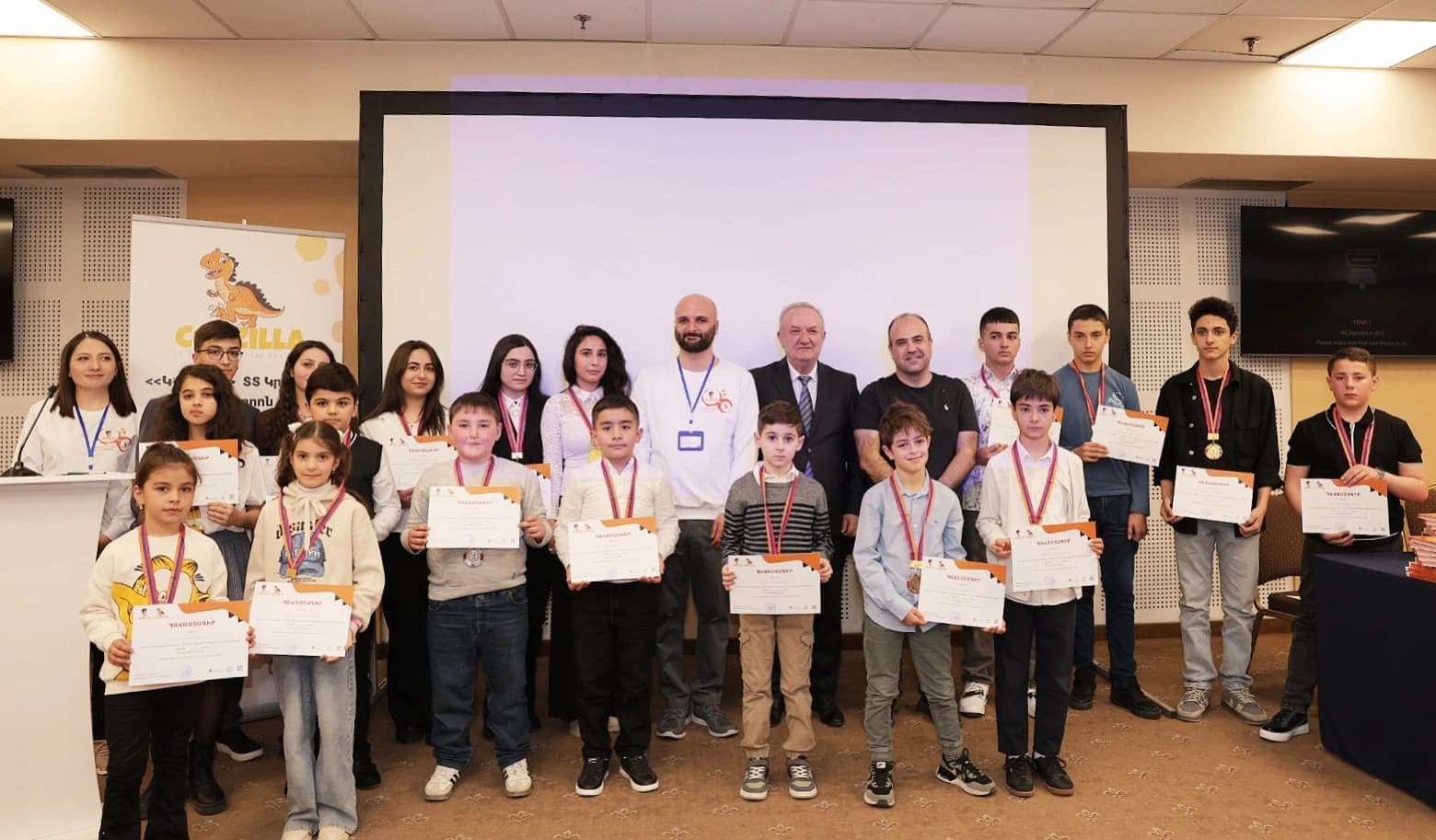
- Codecademy's Debugging Course
- Khan Academy's Introduction to Algorithms
- GitHub Learning Lab – Hands-on tutorials for version control and collaboration
Final Takeaway
Debugging is not just a necessary evil in the world of programming—it’s a gateway to becoming a proficient and thoughtful coder. By embracing debugging techniques, you empower yourself to tackle challenges head-on, transform mistakes into lessons, and ultimately achieve greater success in algorithmic thinking. So, keep debugging, keep learning, and watch your coding skills soar!